Aloha people,
it's the time of the year again where coding elves earn some internet points and learn cool stuff while doing that. Ofc I am talking about advent of code. This year I want to use a recently learned language and re-visit some I haven't used in a while. I also try to pair with some colleagues on the puzzles as I am still on the beach and this are the perfect coding exercises between the cloud provider workshops.
In these years advent of code the story starts with a careless elf who has dropped the sleigh keys into the ocean. Ohhh no! Good that the elves have a submarine ready to go!
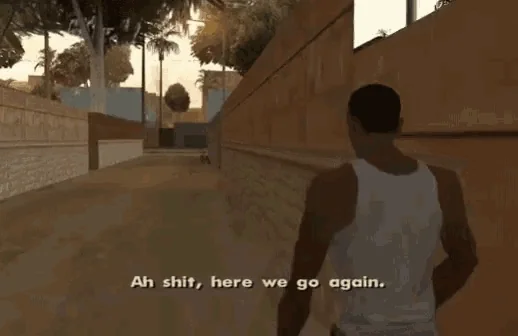
My approach to the first part of the puzzle was rather simple:
- Read in the file as a vector of u32
- Iterate over the measurements and increase a counter when ever there is an increase
- Profit
Interestingly is that it was my first time using IntelliJ to create the Rust project, and it took me no time to get first results. I just wonder whether all unit tests are to be put into the production class itself, for now I am following what I see in the Rust workshops I have visited. Here is the method for part one of the puzzle. We expect a reference from a vector which contain unsigned numbers. I am still a beginner with this language so the decision whether to use i32 or u32 I made simply because all values in the puzzle input were positive.
fn count_increases_of_measurements(measurements: &Vec<u32>) -> u32 {
let mut count_of_increases: u32 = 0;
let mut latest_increased_measurement: &u32 =
measurements.first().unwrap();
for measurement in measurements {
if measurement > latest_increased_measurement {
count_of_increases = count_of_increases.add(1)
}
latest_increased_measurement = measurement;
}
return count_of_increases;
}
Now that solved part one of the puzzle only so that part two could give me a headache, but Rust came to the rescue. The new puzzle is to implement a sliding window over the vector so that we can calculate the sum of it's tuple. Let me visualise this:
199 A
200 A B
208 A B C
210 B C D
200 E C D
207 E F D
240 E F G
269 F G H
260 G H
263 H
The puzzle is to calculate the sum of 199,200,208
and then switching to the next tuple 200,208,210
and so on. I never have seen this kind of problem, and so I was wondering for a short time how to solve this. In the end it's not really hard. You would create slices from the vector and save the sum of them to another vector. I was fiddling around with the vector as Rust showed me this great method windows(n)
. It creates a list of tuples which I could just add up to get the sum. Voilà and so I had solved part two of day one.
fn collect_sum_of_triple_measurements(measurements: &Vec<u32>) -> Vec<u32> {
let triples = measurements.windows(3);
let mut collection_sums:Vec<u32> = Vec::new();
for triple in triples {
let sum = triple.iter().sum();
collection_sums.push(sum);
}
return collection_sums
}
#[derive(Debug)]
pub enum State {
Start,
Transient,
Closed,
}
impl From<&'a str> for State {
fn from(s: &'a str) -> Self {
match s {
"start" => State::Start,
"closed" => State::Closed,
_ => unreachable!(),
}
}
}
That's it for day one so far. Write me in the comments how exited you are!